class: center, middle, inverse, title-slide .title[ # Control Flow ] .subtitle[ ## Mini-Lecture 6 ] .author[ ### Albert Y. Kim ] .date[ ###
SDS 270
2022-09-20
] --- <!-- class: center, inverse, middle # Slack review --- ## CRAN content > I'm wondering what topics of R packages are CRAN material? I was looking at the list of CRAN packages, most of them seem to be statistically inclined. What other topics does CRAN tend to like? -- - CRAN doesn't judge the *content* of your package - It only cares about whether your package is *compliant* -- - CRAN is still maintained by **humans**!! .footnote[https://www.r-project.org/contributors.html] --- ## Test files > Getting an error message when I run the check saying that there's no test files found for my package... any suggestions? -- - You have a `testthat` infrastructure but no actual tests - `usethis::use_test("something")` to create one OR - just ignore the error for now --- ## `NextMethod()` ```r helium <- function(x,...) { UseMethod("helium") } helium.default <- function(x,...) { print("hi") } helium.first <- function(x,...) { message("this is the first method") emoji::emoji("hot") NextMethod() } helium.second <- function(x,...) { message("this is the second method") emoji::emoji("balloon") } ``` --- ## Method dispatch ```r x <- "whatever" class(x) <- c("first", class(x)) class(x) ``` ``` ## [1] "first" "character" ``` -- ```r helium(x) ``` ``` ## this is the first method ``` ``` ## [1] "hi" ``` -- ```r sloop::s3_dispatch(helium(x)) ``` ``` ## => helium.first ## helium.character ## -> helium.default ``` --- ## Method dispatch 2 ```r class(x) <- c("first", "second", class(x)) class(x) ``` ``` ## [1] "first" "second" "first" "character" ``` -- ```r helium(x) ``` ``` ## this is the first method ``` ``` ## this is the second method ``` ``` ## [1] "🎈" ``` -- ```r sloop::s3_dispatch(helium(x)) ``` ``` ## => helium.first ## -> helium.second ## * helium.first ## helium.character ## * helium.default ``` --- ## Recursion? ```r helium.second <- function(x,...) { message("this is the second method") emoji::emoji("balloon") * NextMethod() } ``` -- ```r helium(x) ``` ``` ## this is the first method ``` ``` ## this is the second method ``` ``` ## this is the first method ``` ``` ## [1] "hi" ``` ```r sloop::s3_dispatch(helium(x)) ``` ``` ## => helium.first ## -> helium.second ## -> helium.first ## helium.character ## -> helium.default ``` --> --- class: center, middle, inverse # Control Flow --- ## Vectorized conditionals ```r ifelse(mtcars$am == 1, "man", "auto") ``` ``` ## [1] "man" "man" "man" "auto" "auto" "auto" "auto" "auto" "auto" "auto" ## [11] "auto" "auto" "auto" "auto" "auto" "auto" "auto" "man" "man" "man" ## [21] "auto" "auto" "auto" "auto" "auto" "man" "man" "man" "man" "man" ## [31] "man" "man" ``` ```r case_when( mtcars$am == 1 ~ "man", mtcars$am == 0 ~ "auto" ) ``` ``` ## [1] "man" "man" "man" "auto" "auto" "auto" "auto" "auto" "auto" "auto" ## [11] "auto" "auto" "auto" "auto" "auto" "auto" "auto" "man" "man" "man" ## [21] "auto" "auto" "auto" "auto" "auto" "man" "man" "man" "man" "man" ## [31] "man" "man" ``` -- - See also [`switch()`](https://en.wikipedia.org/wiki/Switch_statement) (not vectorized) --- ## Loops .pull-left[ 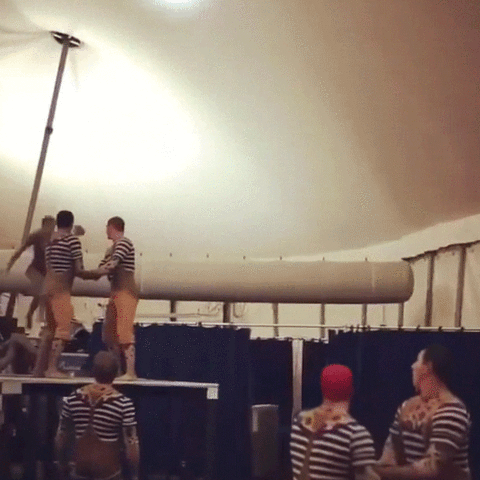 ] .pull-right[ - `for` `\(\subset\)` `while` `\(\subset\)` `repeat` - I've never used a `repeat` loop - I've **very rarely** used a `while` loop - `tidyverse` fans are very opinionated about **never** using `for` loops ] -- - Remember: **R is vector-based**, so many operations don't require loops! --- ## Replace `for` loops with `map()` -- - In general, ```r y <- list() for (i in seq_along(x)) { y[[i]] <- my_fun(i) } ``` -- - Can become ```r y <- map(x, my_fun) ``` -- - shorter, cleaner, **easier to read** -- - more expressive! -- - likely more efficient (makes fewer copies)