class: center, middle, inverse, title-slide .title[ # More about Functions ] .subtitle[ ## Mini-Lecture 9 ] .author[ ### Albert Y. Kim ] .date[ ###
SDS 270
2022-10-20
] --- --- class: center, inverse, middle # More about functions --- ## The dots (...) .pull-left[ - Passing arguments to other functions - Accepting arguments from S3 methods ] .pull-right[ 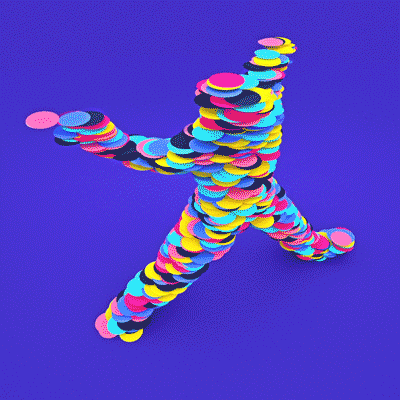 ] --- ## Passing arguments ```r ggplot2::scale_alpha_continuous ``` ``` ## function (..., range = c(0.1, 1)) ## { ## continuous_scale("alpha", "alpha_c", rescale_pal(range), ## ...) ## } ## <bytecode: 0x7fecc6ee3b30> ## <environment: namespace:ggplot2> ``` ```r args(ggplot2::continuous_scale) ``` ``` ## function (aesthetics, scale_name, palette, name = waiver(), breaks = waiver(), ## minor_breaks = waiver(), n.breaks = NULL, labels = waiver(), ## limits = NULL, rescaler = rescale, oob = censor, expand = waiver(), ## na.value = NA_real_, trans = "identity", guide = "legend", ## position = "left", super = ScaleContinuous) ## NULL ``` --- ## You can capture the dots ```r catch_the_dots <- function(...) { list(...) } catch_the_dots(whatever = "hi", "bye") ``` ``` ## $whatever ## [1] "hi" ## ## [[2]] ## [1] "bye" ``` --- ## You can use arguments that a generic function doesn't accept! ```r args(plot) ``` ``` ## function (x, y, ...) ## NULL ``` ```r plot(rnorm(20), rnorm(20), main = "Sweet plot") ``` 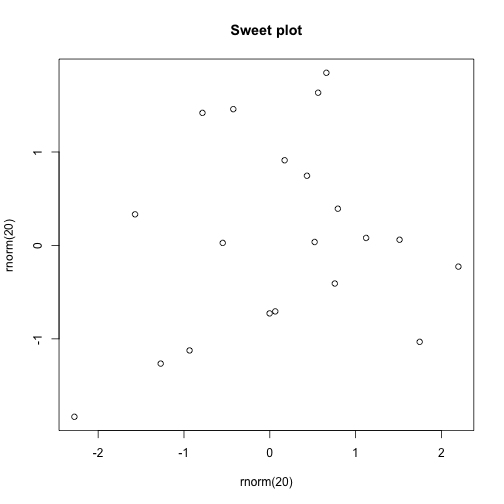<!-- --> --- ## Check arguments ```r body(plot) ``` ``` ## UseMethod("plot") ``` ```r args(plot.default) ``` ``` ## function (x, y = NULL, type = "p", xlim = NULL, ylim = NULL, ## log = "", main = NULL, sub = NULL, xlab = NULL, ylab = NULL, ## ann = par("ann"), axes = TRUE, frame.plot = axes, panel.first = NULL, ## panel.last = NULL, asp = NA, xgap.axis = NA, ygap.axis = NA, ## ...) ## NULL ``` --- ## Return values .pull-left[ - Implicit returns - Watch out for types! - Explicit return with `return()` ] .pull-right[ 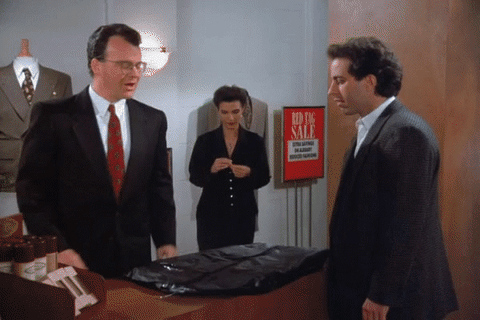 ] --- ## Type problems ```r what_do_i_return <- function(x) { if (x %% 10 == 0) { return(as.character(x)) } else { return(x) } message("This will never get run") } what_do_i_return(100) ``` ``` ## [1] "100" ``` ```r what_do_i_return(53) ``` ``` ## [1] 53 ``` --- ## Function is vectorized, but not appropriately! ```r what_do_i_return(c(100, 53)) ``` ``` ## Error in if (x%%10 == 0) {: the condition has length > 1 ``` --- ## Coercion (Originally on 10/20 Lec 13) ```r map(c(100, 53), what_do_i_return) ``` ``` ## [[1]] ## [1] "100" ## ## [[2]] ## [1] 53 ``` -- ```r map_int(c(100, 53), what_do_i_return) ``` ``` ## Error: Can't coerce element 1 from a character to a integer ``` -- ```r map_chr(c(100, 53), what_do_i_return) ``` ``` ## [1] "100" "53.000000" ``` --- ## Coercion (Corrected 10/25 Lec 14) ```r map_int(c(100, 53), what_do_i_return) ``` is not a good error example because there are two errors: 1. `what_do_i_return(100)` returns `"100"` a character 1. `what_do_i_return(53)` returns `53` a double, not an int -- Instead use `map_dbl()` to test error: ```r map_dbl(c(100, 53), what_do_i_return) ``` ``` ## Error: Can't coerce element 1 from a character to a double ``` -- ```r map_dbl(c(13, 53), what_do_i_return) ``` ``` ## [1] 13 53 ``` --- ## Invisibility - Force visibility with `()` ```r cant_see_me <- function(x) { invisible(x) } cant_see_me("now") ``` -- ```r y <- cant_see_me("later") y ``` ``` ## [1] "later" ``` -- ```r (cant_see_me("now")) ``` ``` ## [1] "now" ```