class: center, middle, inverse, title-slide .title[ # Functionals ] .subtitle[ ## Mini-Lecture 10 ] .author[ ### Albert Y. Kim ] .date[ ###
SDS 270
2022-10-25
] --- class: center, inverse, middle # purrr 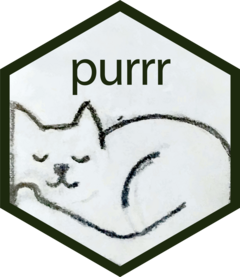 --- ## Highlights .pull-left[ You **need** to know: - `map()` - `map2()` - `pmap()` - `walk()` ] -- .pull-right[ Don't worry as much about: - `modify()` - `imap()` - `reduce()` - `accumulate()` ] --- ## `map()` (is just `lapply()`) .center[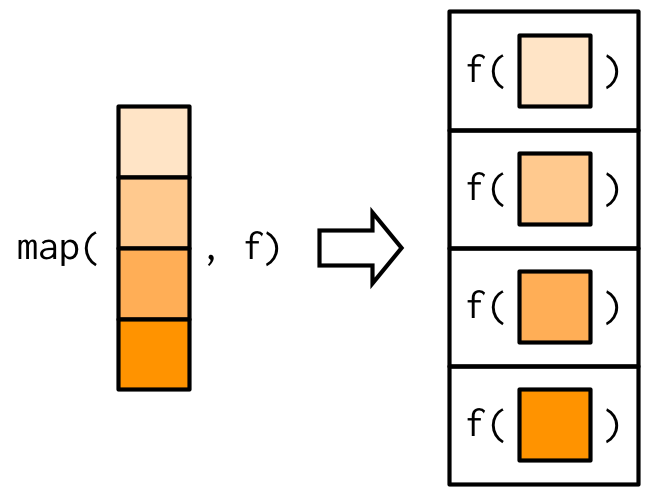] --- ## `map()` with scalar second argument .center[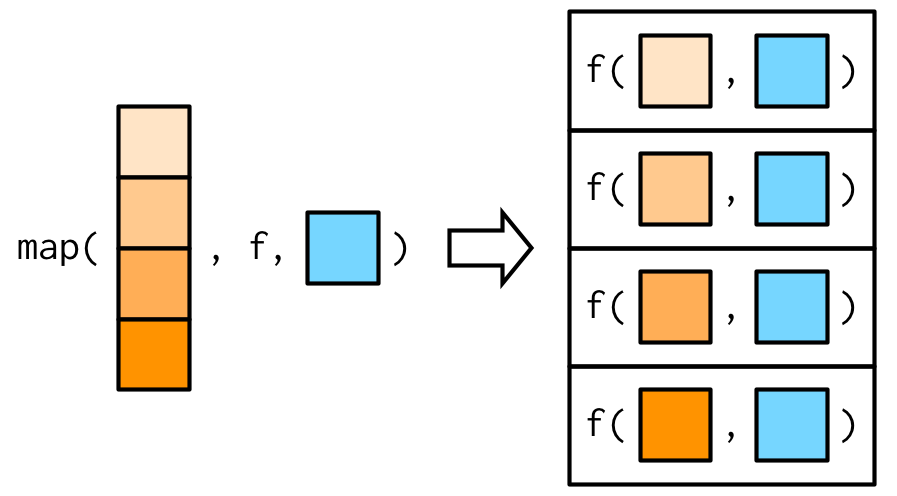] --- ## `map()` with vector second argument .center[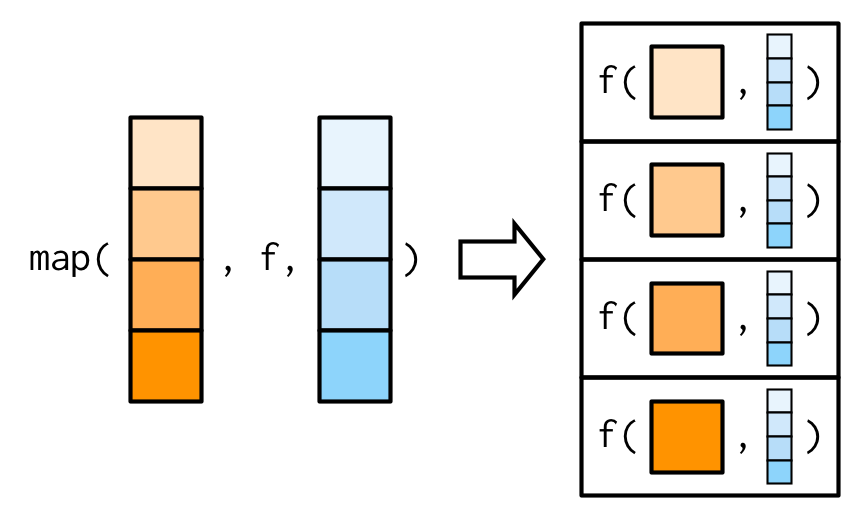] --- ## `map()` examples ```r library(tidyverse) map_int(starwars$films, length) ``` ``` ## [1] 5 6 7 4 5 3 3 1 1 6 3 2 5 4 1 3 3 1 5 5 3 1 1 2 1 2 1 1 1 1 1 3 1 2 1 1 1 2 ## [39] 1 1 2 1 1 3 1 1 1 3 3 3 2 2 2 1 3 2 1 1 1 2 2 1 1 2 2 1 1 1 1 1 1 1 2 1 1 2 ## [77] 1 1 2 2 1 1 1 1 1 1 3 ``` ```r map_chr(mpg, typeof) ``` ``` ## manufacturer model displ year cyl trans ## "character" "character" "double" "integer" "integer" "character" ## drv cty hwy fl class ## "character" "integer" "integer" "character" "character" ``` ```r # infix math operators, like +, - , etc map(1:3, `^`, 2) ``` ``` ## [[1]] ## [1] 1 ## ## [[2]] ## [1] 4 ## ## [[3]] ## [1] 9 ``` --- ## Splitting a `data.frame` into groups\* .footnote[\* This is not in the book!] Option 1: use `group_split()` and `map()`. `group_split()` returns a `list` of `tibble`s ```r mods_split <- mpg %>% * group_split(year) %>% map(~lm(hwy ~ displ + trans, data = .x)) ``` -- Option 2: use `group_by()` and `group_map()`. `group_map()` is like `map()` but on a **grouped** `tibble` ```r mods_by <- mpg %>% group_by(year) %>% * group_map(~lm(hwy ~ displ + trans, data = .x)) ``` --- ## `map2()` with scalar *third* argument 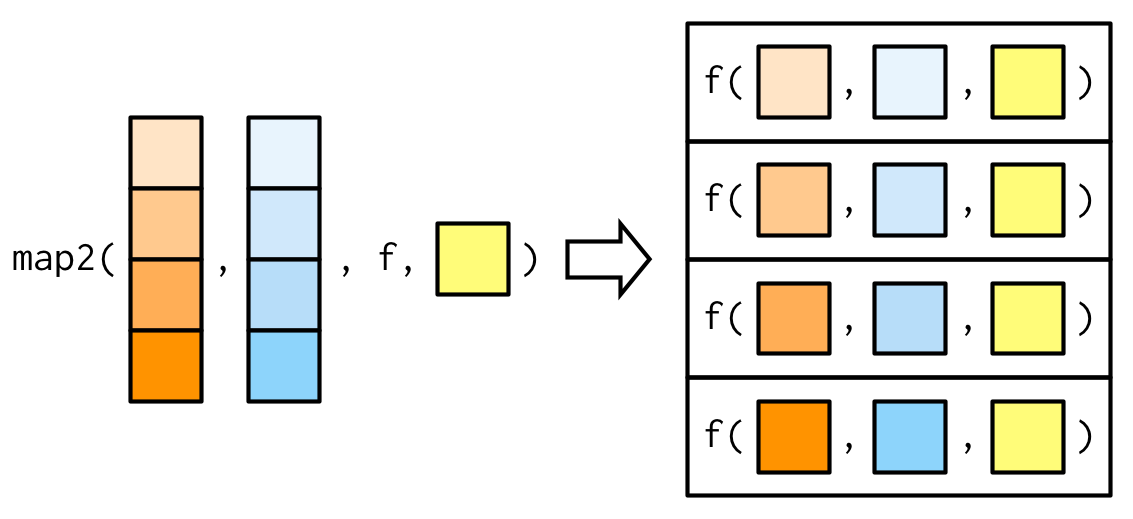 --- ## `map2()` examples ```r map2_lgl( mods_split, mods_by, ~identical(coef(.x), coef(.y)) ) ``` ``` ## [1] TRUE TRUE ``` ```r map2_dbl(1:3, 0:2, `^`) ``` ``` ## [1] 1 2 9 ``` --- ## `pmap()` 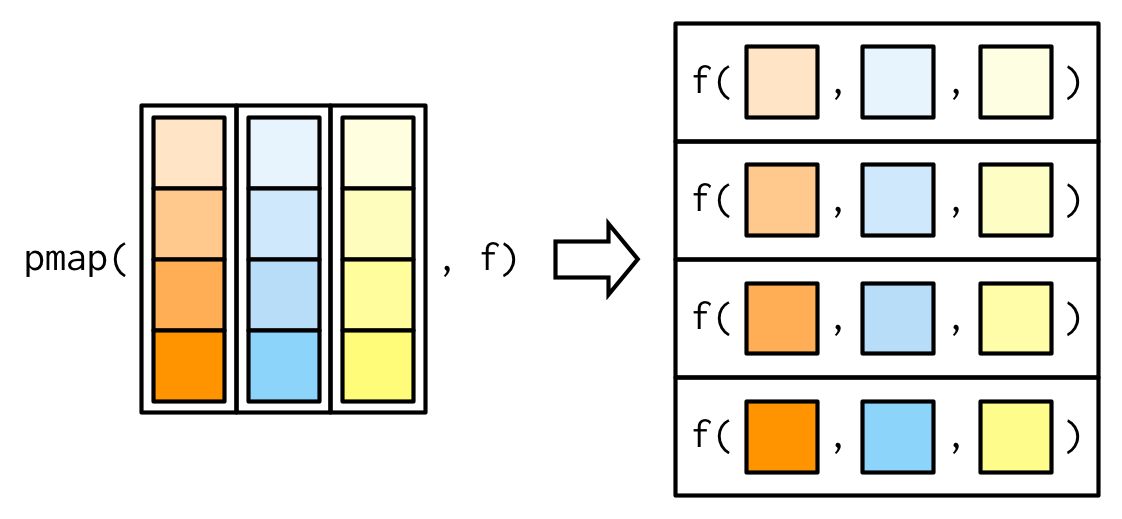 --- ## `pmap()` example ```r params <- tibble( mean = -1:1, sd = c(0.5, 1, 2) ) args(rnorm) ``` ``` ## function (n, mean = 0, sd = 1) ## NULL ``` ```r pmap(params, rnorm, n = 1000) %>% map_dbl(mean) ``` ``` ## [1] -0.96089539 0.01771944 1.06744975 ```